Attribute values
This section presents the possible distributions that can be assigned to the attributes of the simulation objects. A distribution is defined in the form of a list. The first element of the list is always a string of length 1, which serves as an identifier for the different distributions. The remaining attributes define the specific distribution parameters.
Distribution |
Identifier |
Parameters |
---|---|---|
/ |
/ |
|
f |
[“f”, \(\nu\) ] |
|
b |
[“b”, p ] |
|
i |
[“i”, n , p ] |
|
n |
[“n”, \(\mu\) , \(\sigma\) ] |
|
u |
[“u”, a , b ] |
|
p |
[“p”, \(\lambda\) ] |
|
e |
[“e”, \(\beta\) ] |
|
l |
[“l”, \(\mu\) , \(\sigma\) ] |
|
c |
[“c”, n ] |
|
t |
[“t”, n ] |
User defined
If the desired distribution is not predefined, then the user can define custom distribution functions. Such a function is defined in the global scope of the function input file. The function’s name later serves as an identifier and thus must have length 1 and not intersect with the predefined ones. Since the return value of such a function is assigned to the attributes, the return type must be int or float. However, the defined function can have any number of arguments of any type. In the distribution list, the arguments of this function are then passed in the same order.
Example:
The task is to define the following distribution function, which is not predefined, and then assign it to an attribute.
It is possible to define a function that uses the values shown. Alternatively, a general function is defined that can be used to map other arbitrary discrete distributions. For this purpose, x is used as a free identifier and choice from numpy.random as distribution. The attributes of the function x are two lists that contain the probabilities and discrete values.
from numpy.random import choice
def x(values, probabilities) -> Union[int, float]:
return choice(a=values, p=probabilities)
Now, this distribution needs to be added to the attribute.
"attr_1": ["x",[0.9,1.8,2.9,6],[0.5,0.25,0.125,0.125]]
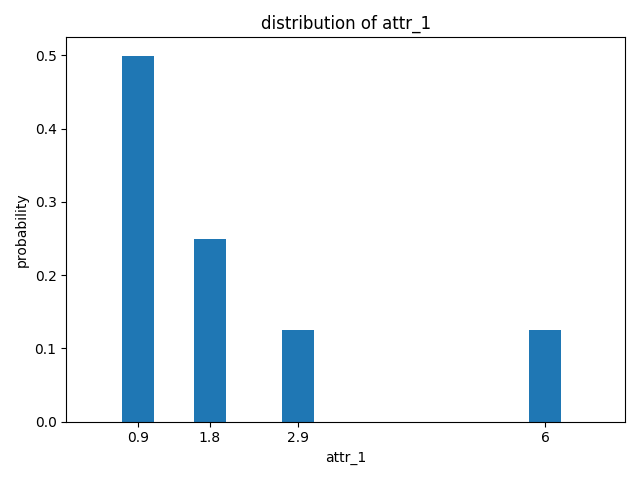
Note
User-defined distributions are not checked by the inspector.
Fixed
The identifier f indicates a fixed attribute value. The second parameter of the list specifies the fixed value \(\nu\) that the attribute takes. Like all other attributes, the types int and float are possible. Distribution:
Distribution:
Example:
"prob_of_failure": ["f",4.34]
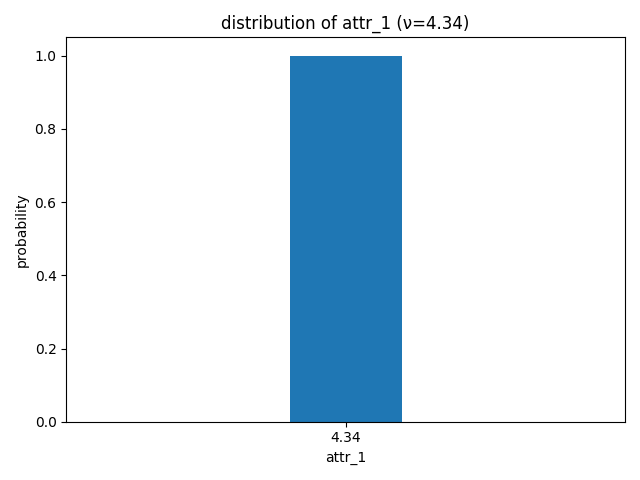
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
f |
|
Additional parameter |
\(\nu\) |
Value |
Exceptions |
InvalidFormat |
List does not have length 2 |
InvalidType |
\(\nu\) is not of type int or float |
Binary
The identifier b indicates a binary attribute value. The second element of the list is the probability p that the attribute takes the value 1. B(k,p) indicates the probability that an attribute takes the values k, given probability p.
Distribution:
Example:
"attr_1": ["b",0.2]
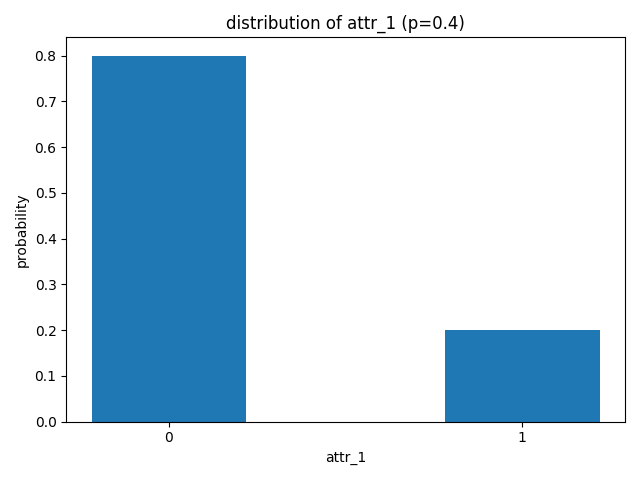
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
b |
|
Additional parameter |
p |
Success probability |
Exceptions |
InvalidFormat |
List does not have length 2 |
InvalidType |
p is not of type int or float |
|
InvalidValue |
p is not between 0.0 and 1.0 |
Binomial
The identifier i indicates a binomial attribute value. The second list element is the number of trails n, while the third is the success probability p. B(k,p) indicates the probability that an attribute takes the values k, given probability p and the number of trails n.
Distribution:
Example:
"attr_1": ["i",5,0.4]
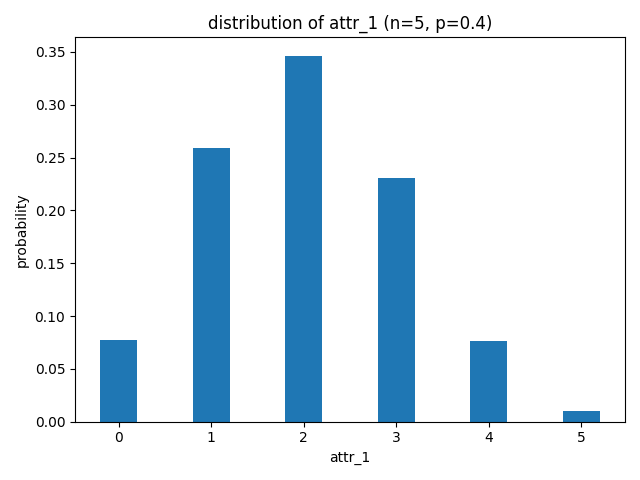
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
i |
|
Additional parameter |
n |
Number of trails |
Additional parameter |
p |
Success probability for each trail |
Exceptions |
InvalidFormat |
List does not have length 2 |
InvalidType |
n is not of type int |
|
p is not of type int or float |
||
InvalidValue |
n is not greater than zero |
|
p is not between 0.0 and 1.0 |
Normal
The identifier n indicates a normally distributed attribute. The second list entry corresponds to the mean \(\mu\) and the third to the standard deviation \(\sigma\).
Distribution:
Example:
"attr_1": ["n",4,2.5]
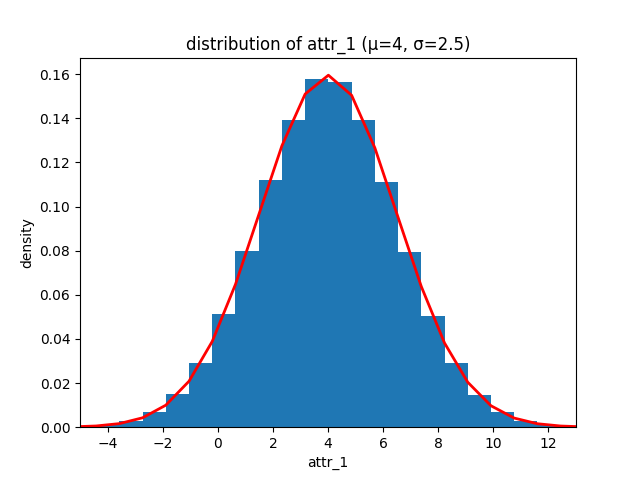
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
n |
|
Additional parameter |
\(\mu\) |
Mean |
\(\sigma\) |
Standard deviation |
|
Exceptions |
InvalidFormat |
List does not have length 3 |
InvalidType |
\(\mu\) or \(\sigma\) is not of type int or float |
|
InvalidValue |
\(\sigma\) is smaller than zero |
Uniform
The identifier u indicates a uniform distributed attribute. The second list parameter a is the lower limit, while the third b sets the upper interval limit. The limits can be integers or floating-point numbers.
Distribution:
Example:
"attr_1": ["u",1,2.34]
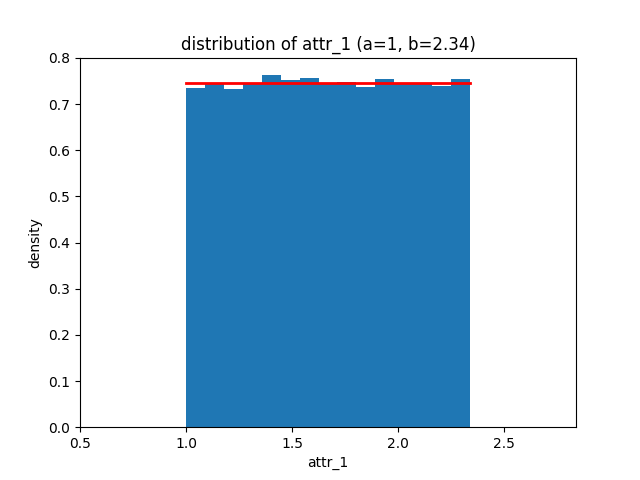
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
u |
|
Additional parameter |
a |
lower bound |
a |
upper bound |
|
Exceptions |
InvalidFormat |
List does not have length 3 |
InvalidType |
a or b is not of type float or int |
|
InvalidValue |
a is greater or equal b |
Poisson
The identifier p indicates a Poisson-distributed attribute. The second list entry determines the rate \(\lambda\), which must be type float or int and greater than or equal to zero. \(P(k,\lambda)\)lambda`.
Distribution:
Example:
"attr_1": ["p",2.1]
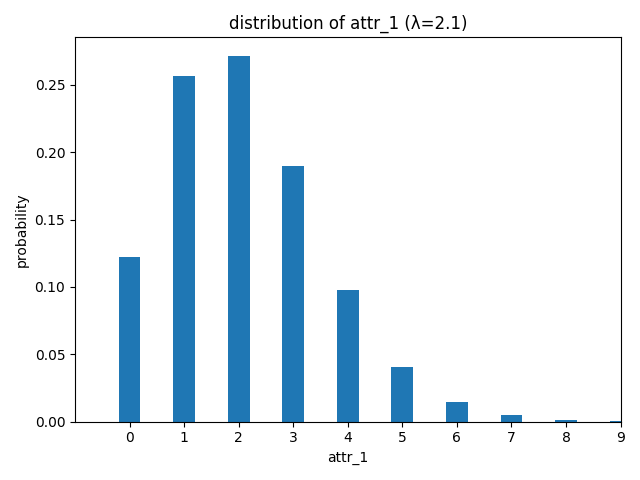
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
p |
|
Additional parameter |
\(\lambda\) |
Rate |
Exceptions |
InvalidFormat |
List does not have length 2 |
InvalidType |
\(\lambda\) is not of type float or int |
|
InvalidValue |
\(\lambda\) is less than zero |
Exponential
The identifier e indicates an exponential distributed attribute. The second list element is the scale \(\beta\), which can be a positive floating-point number.
Distribution:
Example:
"attr_1": ["e",2.5]
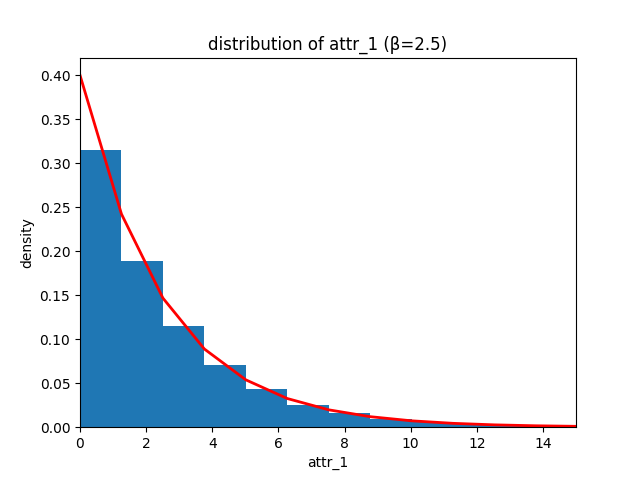
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
e |
|
Additional parameter |
\(\beta\) |
Scale |
Exceptions |
InvalidFormat |
List does not have length 2 |
InvalidType |
\(\beta\) is not of type float or int |
|
InvalidValue |
\(\beta\) is less or equal to zero |
Note
Often the exponential function is also defined by the rate \(\lambda=\frac{1}{\beta}\), instead of the scale \(\beta\). For more information see numpy.random.exponential.
Lognormal
The identifier l indicates a lognormal distributed attribute. The second list entry corresponds to the mean \(\mu\) and the third to the standard deviation \(\sigma\); \(\mu\) and \(\sigma\) must be of type int or float, while \(\sigma\) must also be greater than or equal to zero.
Distribution:
Example:
"attr_1": ["l",0,0.5]
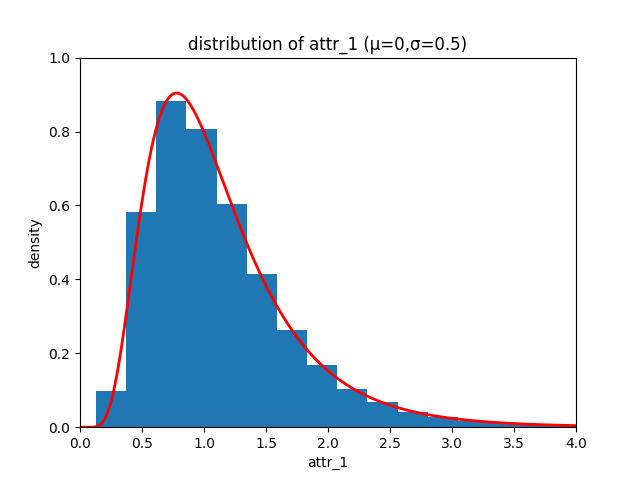
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
l |
|
Additional parameter |
\(\mu\) |
Mean |
\(\sigma\) |
Standard deviation |
|
Exceptions |
InvalidFormat |
List does not have length 3 |
InvalidType |
\(\mu\) or \(\sigma\) is not of type float or int |
|
InvalidValue |
\(\sigma\) is less than zero |
Chisquare
The identifier c indicates a chi-square distributed attribute. The second list entry determines the degrees of freedom n, which must be a positive floating-point or integer.
Distribution:
Example:
"attr_1": ["c",2]
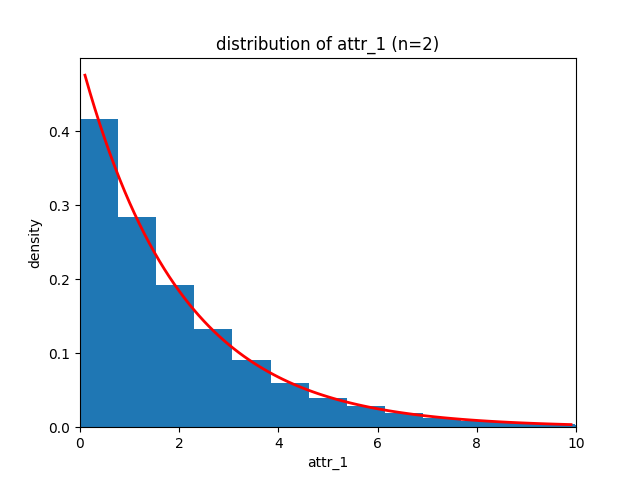
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
c |
|
Additional parameter |
n |
Degrees of freedom |
Exceptions |
InvalidFormat |
List does not have length 2 |
InvalidType |
n is not of type float or int |
|
InvalidValue |
n is less than or equal to zero |
Student-t
The identifier t indicates a student-t distributed attribute. The second list entry determines the degrees of freedom n, which must be a positive floating-point or integer.
Distribution:
Example:
"attr_1": ["t",4]
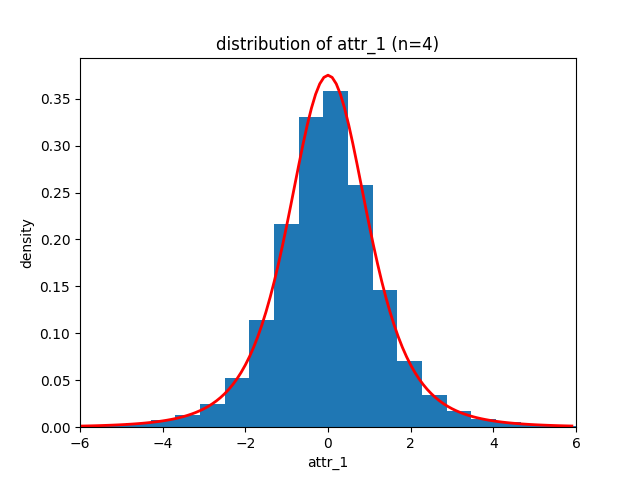
Overview:
Value |
Explanation |
|
---|---|---|
Identifier |
t |
|
Additional parameter |
n |
Degrees of freedom |
Exceptions |
InvalidFormat |
List does not have length 2 |
InvalidType |
n is not of type float or int |
|
InvalidValue |
n is less than or equal to zero |